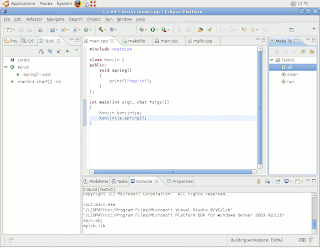
Now and then people I work with ask me for a compiled library of some project for the Windows platform. As I dislike working on Windows, I use a MinGW crosscompiler on my GNU/Linux system to compile the code and thus am able to give them what they want.
Unfortunately, AFAIK the MinGW compiler can't generate debugging symbols compatible with Microsoft's compiler, meaning that although they can use the libraries I provide, they can't step through the code using Microsoft's debugger. Which is really annoying.
So, once in a while I figured it might be interesting to get the Visual C++ compiler working from within my GNU/Linux system. This way I would be able to compile everything with the Microsoft compiler and generate compatible debugging info. And as Microsoft has made the compiler free (as in beer not freedom), it's becoming more and more interesting.
I've tried this several times and in the end created a little script to make it easier to run the VC++ compiler. Afterwards I reported it to the Wine AppDB and although it seemed to work fine, I never really started using it, except for some experimentation.
Today, I was trying to figure out how to add support for the Intel C++ compiler to Eclipse/CDT, when I noticed that CDT plugins were already available in the Intel C++ compiler package... So, as I really wanted to play a bit with CDT, I figured I might as well have a look at how to integrate Microsoft's compiler in Eclipse/CDT.
The easiest way to have something workable is using the Eclipse/CDT GNU Make builder. And that's why I started creating a simple makefile which allows me to build Windows executables and libraries using GNU Make on my Ubuntu system. Eclipse/CDT already includes a VC++ output parser, so that part looks rather nicely integrated. The errors and warnings VC++ outputs, get parsed and added to a list of errors on which you can click, which will take you to the corresponding faulty line of code.
So, as I said, I had another go and created a little Makefile to make it a bit easier to create DLL's from GNU/Linux.
Basically, the only thing you need to do before this Makefile works, is install Microsoft Visual C++ Express on your Windows partition, and afterwards copy the followings directories into your Wine directory $HOME/.wine/drive_c/Program Files:
- c:/Program Files/Microsoft Visual Studio 8/VC/include
- c:/Program Files/Microsoft Visual Studio 8/VC/lib
- c:/Program Files/Microsoft Visual Studio 8/VC/bin
- c:/Program Files/Microsoft Platform SDK for Windows Server 2003 R2/Include
- c:/Program Files/Microsoft Platform SDK for Windows Server 2003 R2/Lib
You can do this as follows:
mkdir -p $HOME/.wine/drive_c/Program\ Files/Microsoft\ Visual\ Studio\ 8/VC
mkdir -p $HOME/.wine/drive_c/Program\ Files/Microsoft\ Platform\ SDK\ for\ Windows\ Server\ 2003\ R2/
cp -r /mnt/windows/Program\ Files/Microsoft\ Visual\ Studio\ 8/VC/{include,bin,lib} $HOME/.wine/drive_c/Program\ Files/Microsoft\ Visual\ Studio\ 8/VC
cp -r /mnt/windows/Program\ Files/Microsoft\ Platform\ SDK\ for\ Windows\ Server\ 2003\ R2/{Include,Lib} $HOME/.wine/drive_c/Program\ Files/Microsoft\ Platform\ SDK\ for\ Windows\ Server\ 2003\ R2/
The makefile:
MSVC=c:/Program\ Files/Microsoft\ Visual\ Studio\ 8/VC/
MSSDK=c:/Program\ Files/Microsoft\ Platform\ SDK\ for\ Windows\ Server\ 2003\ R2/
INCLUDE=/I${MSVC}include /I${MSSDK}Include
LDFLAGS=/LIBPATH:${MSVC}lib /LIBPATH:${MSSDK}Lib
WINE=/opt/wine-dev/bin/wine
CXX=${WINE} ${MSVC}/bin/cl.exe
EXECUTABLE=main.exe
all: ${EXECUTABLE}
%.obj : %.cpp
$(CXX) /c $(INCLUDE) $<
%.lib : %.obj
${CXX} $^ /link /DLL /out:${@:.lib=.dll} ${LDFLAGS}
%.exe : %.obj
${CXX} $^ /link ${LDFLAGS}
mylib.lib: mylib.obj
${EXECUTABLE}: main.obj mylib.lib
clean:
-rm *.obj *.exe *.dll *.lib *.exp
run:
@${WINE} ${EXECUTABLE}
And, finally, there's a screenshot showing Eclipse 3.3.2 with CDT 4.0.3 and Visual C++ 8.0's compiler (version 14.0).
Updated: 2008/04/28 T 09:53.